
Qoddi automatically builds your Go app from your code.
In order to do that, Qoddi uses buildpacks to detect and build your app automatically.
You can fork or build this repository to get started with Go on Qoddi
Before Building your app
To be able to build your app, Qoddi needs several files to be placed inside the root directory of your app :
go.mod
Procfile
(see below)
Note: This tutorial focuses on building a Go app using Go Modules
Create your app on Qoddi
Create a new app and link it with your repository using SSH keys.
Read this article for a detailed procedure or check this video :
go.mod
The go.mod
file determines the version of Go your app is using, manages the packages and everything your app needs to run :
module github.com/flashdriveio/getting-started-with-golang
// +qoddi goVersion go1.12
go 1.12
require github.com/lib/pq v1.10.0
Note: the // +qoddi goVersion goX.xx
line is mandatory for the builder to respect the Go Version or the default version will be used. See below on how to override the Go Version using an env variable.
The builder is compatible with the official Heroku buildpack for Go, the instruction // +heroku goVersion go1.16
will be also respected by the builder.
Overriding the Go Version
The builder will always use the last release of the version specified inside the go.mod file (example go1.12 will use the 1.12.17 or newer version).
The specific Go version used by the builder can be overridden by setting an ENV Variable GOVERSION
:

Make sure to rebuild your app after adding/replacing this env variable.
The list of available Go Version is available here.
Passing a Symbol to the linker (and optional string)
Qoddi supports the Go linker’s ability (-X symbol value) to set the value of a string at link time. This can be done by setting $GO_LINKER_SYMBOL
and $GO_LINKER_VALUE
in the application’s config before pushing code. If $GO_LINKER_SYMBOL
is set, but $GO_LINKER_VALUE
isn’t set then $GO_LINKER_VALUE
defaults to $SOURCE_VERSION
.
This can be used to embed the commit SHA, or other build-specific data directly into the compiled executable.
Other Builder Behavior
Various aspects of the build can be controlled via several application environment variables. Below is a list of the vars that are not covered elsewhere in this doc and what they control:
CGO_CFLAGS
,CGO_CPPFLAGS
,CGO_CXXFLAGS
andCGO_LDFLAGS
: These are the standard CGO flags as described here.GOPROXY
,GOPRIVATE
, andGONOPROXY
: These and the standard environment variables that configure use of module proxies as described here.GOVERSION
: Provides an override to the go version to use.GO_INSTALL_PACKAGE_SPEC
: Overrides the package spec passed togo install
with this value. This is useful when you have a large repo, but only want to compile a sub section of it.GO_INSTALL_TOOLS_IN_IMAGE
: When set totrue
, installs the go tool chain into the slug at$HOME/.heroku/go
, set’s$GOROOT=$HOME/.heroku/go
and includes$GOROOT/bin
in your$PATH
. Normally the go tools are not installed in the slug. This increases the slug size by about 81MB.GO_SETUP_GOPATH_IN_IMAGE
: When set totrue
$GOPATH=$HOME
is set and user code is placed in the appropriate location inside of the$GOPATH
(e.g.$GOPATH/src/github.com/heroku/go-getting-started
). When the dyno starts the working directory will be set to root of the user code. Normally the buildpack keeps user code in$HOME
. This is most useful when used in conjunction withGO_INSTALL_TOOLS_IN_IMAGE=true
so that there is a fully functioning go workspace and tool chain inside of the dyno.
Each of the above environment variables need to be set on an application before pushing code and code must be pushed after these config vars are set for them to take effect.
Procfile
The Procfile informs the engine on the type of app you are running (web or worker) and prepares the infrastructure accordingly. Make sure to use the same server type as during the app creation (web or worker) or you will need to manually alter the network settings of the app.
The Procfile basically contains the type of app and the initial script to run when the app starts :
web: bin/getting-started-with-golang
Make sure the command matches the app name setup inside the go.mod file
Add Go Packages
If go.mod file is present inside the app root folder, Qoddi will build the image with additional packages. The proper way to use Go packages is described here.
Default Behavior
By default, your Go app is routed to the 8000 port of the container. If you need to change the instance port of your app you can do it from the app settings page.
Note: you can set up any container port but Qoddi will only route 80/443 traffic to your app. Qoddi’s apps are not reachable from the outside of Qoddi on any other port.
Sessions handling
Since Qoddi supports dynamic local storage (called Virtual Disks) that are shared between the same app even if it’s working on different hosts (members of the same stack), you can create a virtual drive inside the app settings to store that information.
You can also use the Gorilla toolkit to store the sessions on the client-side. Make sure to create the environment variables SESSION_AUTHENTICATION_KEY
and SESSION_ENCRYPTION_KEY
before you build your app.
Launch a Console Command
Please check this article on how to launch a command directly to your running app. This can be used to perform database migrations, launch local scripts, or perform database backups.
Ephemeral Disk
Contrary to Marketplace apps that can use Qoddi’s virtual disks features to store and retrieve persistent data, apps that are created from code use an immutable file system.
You can write data locally, for instance, to process files temporarily during your application life, but the data written into your app’s disk is not persistent and gets wiped out after your app restart.
All apps restart at least once every 24 hours, to apply a new setting or to deploy a new build.
To store data and keep it persistent you can use external services like Amazon S3 or Wasabi, an S3 compatible storage service with very affordable pricing.
Add a domain name
Please check this article for a detailed method on how to use your domain name with Qoddi apps.
Define environment variables
Note: Environment variables set in your app settings are injected during the build process. If the build of your app needs to use an env variable make sure to set it before requesting the build.
Qoddi lets you externalize the configuration of your app : the Qoddi’s cluster will automatically injects the data when the app start or restart.
Environment variables can include any external data your app needs to run like external resources, databases addresses, encryption keys, etc…
To add an environment variable, access your app settings and click “Add ENV Variable” :

Enter the key (like DATABASE_USER) and the value and click “Add”. Qoddi will automatically add your environment variable and restart your app after a one-minute delay (to let you add more env variables if necessary without restarting the app each time a new variable is added).
Provision a database
Qoddi Marketplace includes all popular datastore engines including Mysql, Postgres, MongoDB, and Redis.
To add a database to your app, click on Marketplace and select the database you want to run, then create the new app database inside the same stack as your Ruby app.
Once the app is running, visit the database app’s settings and retrieve the “internal name” :
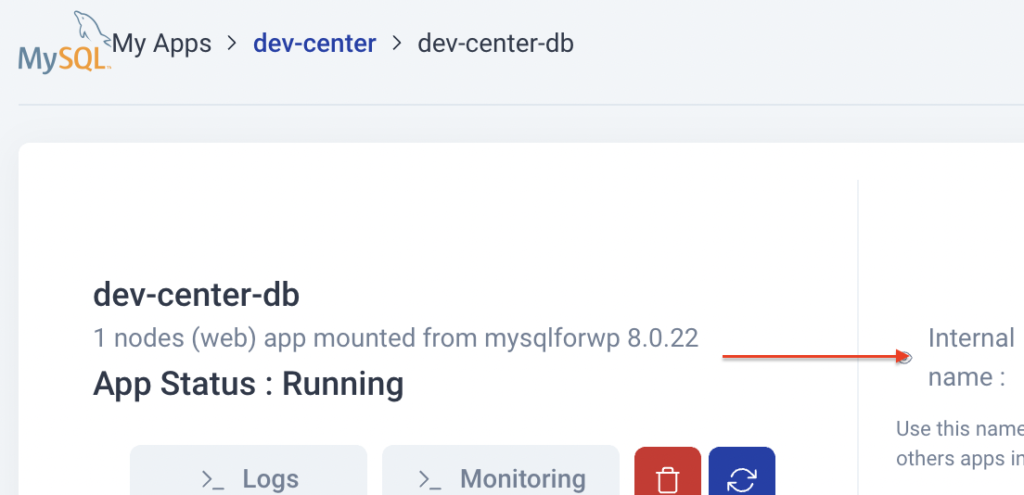
Use this internal name as you would use a server address, for instance for MySQL this name replaces the usual “localhost”. Some scripts require you to add the database port at the end of the server address. Qoddi uses a common port for each database software, for MySQL it will be 3306 and you can write it like that inside your scripts: internal-name:3306
Postgres usually uses credentials sent inside the login URL, like that : postgres://<user>:<password>@<internalname>:5432/<database>
The login and password information is available inside the Environment Variables of the database app.
Note: Qoddi’s datastores run on a private and encrypted network. Therefore SSL support is deactivated for all datastores available on Qoddi. Ensure that your code doesn’t require using SSL before deploying your app.
Reach your app from another app
Inside your app stack, you can reach any other Qoddi app member of this stack on any port. Qoddi automatically routes the traffic internally and opens the appropriate port. By opposition, your app is only reachable on ports 80/443 from outside of Qoddi.
To reach your app internally, retrieve the internal name from your app settings page :
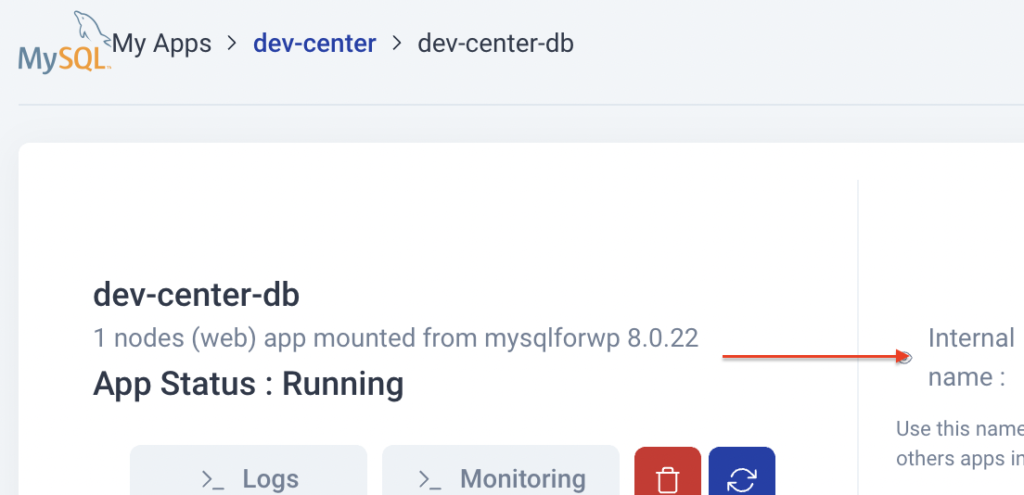
This name can be used to reach your app from another app inside the same stack. Use it in any form that works with your script :
http://<internalname>
<internalname>
http://internalname>:port
Note: https:// is not available, all the traffic inside apps is already encrypted by the cluster.
Encryption
Qoddi uses at rest encryption for the app virtual disks, build images, and encrypted transport from inside the cluster. To make sure the traffic is encrypted between your app and the browser of your users you can set up SSL certificates for any domain name connected to the app (including the Qoddi’s default domain name).
Scale your App
From your app settings you can scale your app vertically and horizontally: by upgrading the app size (refer to our pricing for app sizes details) or by adding more nodes.
Additional nodes will run another copy of your app inside another Qoddi server located inside the same cluster (same geographical location). Traffic is automatically sent to the least occupied node by Qoddi’s load balancer.
Was this helpful?
6 / 3